For Web Apps
The OAuth 2.0 Authorization Code Grant is suitable for web applications that can maintain a secret--this requires server-side implementation on your part.
This is the recommended OAuth 2.0 flow for most integrations, since the refresh_token can be used to extend authorization beyond 24 hours (unlike the Implicit Grant Flow), greatly improving user experience.
Overview
- Redirect browser to the /oauth2 endpoint
- User will be prompted to accept your OAuth 2.0 request
- We redirect browser to your application's redirect_uri; parse Authorization Code from the URL query string
- POST the Authorization Code, along with your client credentials, to our /token endpoint. This must be done from your server--your client_secret should never be exposed to a browser.
- We respond with a JSON containing access_token + refresh_token
- Refresh access_token when it expires
OAuth 2.0 Endpoint
URL
https://secure.join.me/api/public/v1/auth/oauth2
Method
GET
Open in full window or popup -- not iframeable
Query Parameters
Parameter | Value | Description |
---|---|---|
response_type | code | Required. Indicates which OAuth 2.0 workflow the application is requesting. Auth server will return Authorization Code in URL query string. |
client_id | Your application's API Key | Required. |
scope | Accepted values: | Required. Space-delimited list of requested authorization privileges |
redirect_uri | The value entered in your application's Callback URL field | Required. The URL the authorization server redirects the user to once they accept or decline the OAuth 2.0 request. |
state | any string | Optional, but strongly recommended. A value which will be passed through to the redirect_uri;, used to protect against possible CSRF attacks (learn more here) |
Note: When the initial request is made, parameters are validated before the user is directed to log in. Any missing parameters or parameters in invalid format will be reported via an error page. If an error occurs during the subsequent OAuth 2.0 flow, the user agent is redirected to the URL defined in the redirect_uri parameter with the error description in the URL fragment.
Example Request URL
https://secure.join.me/api/public/v1/auth/oauth2?client_id={api_key}&scope=scheduler%20start_meeting&redirect_uri=https://domain.com/callback&state=ABCD&response_type=code
Request OAuth 2.0 Permission
The user will be presented with the following OAuth 2.0 permission prompt:
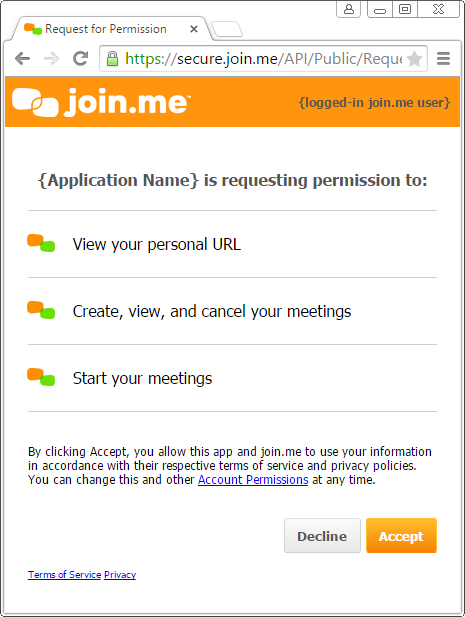
Authorization Code Response
Parameter | Description |
---|---|
code | An Authorization Code that can be exchanged for an access_token via the /token endpoint. This code expires after 1 minute. |
state | A state value is returned only if the application passed the state parameter for CSRF protection. |
error | Indicates an error occurred during the request permission flow. If the user explicitly denies your application's request, this error will be set to "access_denied". |
Response will be provided via the URL query string. It is recommended that this is parsed server-side, since the Authorization Code must be exchanged for an access_token via a server-to-server API request.
Example Success Response
https://example.com/redirect.html?code={Authorization Code}&state={your state}
Example Error Response
https://example.com/redirect.html?error=access_denied&state={your state}
Token Endpoint
URL
https://secure.join.me/api/public/v1/auth/token
Method
POST
This request must come from your server to preserve client_secret
Parameters
These parameters should be JSON or x-www-form-urlencoded, in the body of the POST request
Parameter | Description |
---|---|
client_id | Required. Your application's API Key |
client_secret | Required. Your application's Secret. As the name implies, this should be securely stored on your server, and never passed to a frontend. |
code | Required. The Authorization Code you received after sending a join.me user through the /oauth2 request permission flow with response_type=code. Used to exchange an Authorization Code for an access_token. |
redirect_uri | Required. The same redirect_uri you used in for the /oauth2 call. This is a validation check only--we will respond directly to your HTTP request, not to the redirect_uri. |
grant_type | Required. As per OAuth 2.0 spec, value must be: authorization_code |
Example Request
Request
{
"client_id": "qwer1234",
"client_secret": "asdf5678",
"code": "zxcv90",
"redirect_uri": "https://example.com/redirect",
"grant_type": "authorization_code"
}
Example Response
{
"access_token": "qwer1234",
"token_type": "bearer",
"return_type": "json",
"refresh_token": "asdf5678"
}
Refresh
To refresh an access_token, you'll call the same /token endpoint, but with slightly different parameters. You will need to refresh your tokens when you receive an HTTP 401 response from an API endpoint (indicating the access_token is expired).
Note: Your refresh_token is invalidated during this flow--successful refresh will create a new access_token + refresh_token pair, and you should use this new refresh_token for the next refresh request.
The refresh_token, like client_secret, is also confidential--do not expose this to the browser.
URL
https://secure.join.me/api/public/v1/auth/token
Method
POST
This request must come from your server to preserve client_secret + refresh_token
Parameters
These parameters should be JSON or x-www-form-urlencoded, in the body of the POST request
Parameter | Description |
---|---|
client_id | Required. Your application's API Key |
client_secret | Required. Your application's Secret. As the name implies, this should be securely stored on your server, and never passed to a frontend. |
refresh_token | Required. The current refresh_token corresponding to an expired access_token. This refresh_token is invalidated during this flow (successful refresh will create a new access_token + refresh_token pair). |
grant_type | Required. As per OAuth 2.0 spec, value must be: refresh_token |
Example Request
{
"client_id": "qwer1234",
"client_secret": "asdf5678",
"refresh_token": "zxcv90",
"redirect_uri": "https://example.com/redirect",
"grant_type": "refresh_token"
}
Example Response
{
"access_token": "qwer1234",
"token_type": "bearer",
"return_type": "json",
"refresh_token": "asdf5678",
"scope": "user_info scheduler start_meeting"
}