For JavaScript-Only Apps
The OAuth 2.0 Implicit Grant Flow is suitable for JavaScript-only web applications that cannot maintain a secret (i.e. all code is client-side). This flow is also a good option if you want to quickly prototype with the join.me API.
The major drawback is that access_tokens will expire every 24 hours, with no method of refreshing; you will have to prompt users to re-accept OAuth permissions every day.
Overview
- Redirect browser to the /oauth2 endpoint
- User will be prompted to accept your OAuth 2.0 request
- We redirect browser to your application's redirect_uri; parse access_token from theURL fragment
OAuth 2.0 Endpoint
URL
https://secure.join.me/api/public/v1/auth/oauth2
Method
GET
Open in full window or popup -- not iframeable
Query Parameters
Parameter | Value | Description |
---|---|---|
response_type | token | Required. Indicates which OAuth 2.0 workflow the application is requesting. Auth server will return access_token in URL fragment. |
client_id | Your application's API Key | Required. |
scope | Accepted values: | Required. Space-delimited list of requested authorization privileges |
redirect_uri | The value entered in your application's Callback URL field | Required. The URL the authorization server redirects the user to once they accept or decline the OAuth 2.0 request. |
state | any string | Optional, but strongly recommended. A value which will be passed through to the redirect_uri;, used to protect against possible CSRF attacks (learn more here) |
Note: When the initial request is made, parameters are validated before the user is directed to log in. Any missing parameters or parameters in invalid format will be reported via an error page. If an error occurs during the subsequent OAuth 2.0 flow, the user agent is redirected to the URL defined in the redirect_uri parameter with the error description in the URL fragment.
Example Request URL
https://secure.join.me/api/public/v1/auth/oauth2?client_id={api_key}&scope=scheduler%20start_meeting&redirect_uri=https://domain.com/callback&state=ABCD&response_type=token
Request OAuth 2.0 Permission
The user will be presented with the following OAuth 2.0 permission prompt:
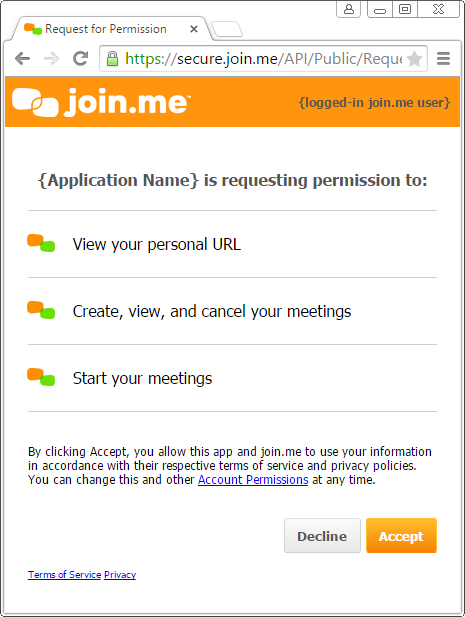
Response
Parameter | Description |
---|---|
access_token | The user's random OAuth 2.0 bearer token |
expires_in | The access_token's time-to-live |
state | A state value is returned only if the application passed the state parameter for CSRF protection. |
error | Indicates an error occurred during the request permission flow. If the user explicitly denies your application's request, this error will be set to "access_denied". |
The response will be provided in the URL fragment, and must be parsed via JavaScript on the redirect_uri page. Be careful with the access_token, because this token grants you access to act on the join.me user’s behalf: it should be stored securely, and only ever transferred over a secure (HTTPS) connection.
Example Success Response
https://example.com/redirect.html#access_token={token}&token_type=bearer&expires_in=1440&state={your state}
Example Error Response
https://example.com/redirect.html#error=access_denied&state={your state}
Example JavaScript to parse URL Fragment
var fragmentString = location.hash.substr(1);
var fragment = {};
var fragmentItemStrings = fragmentString.split('&');
for (var i in fragmentItemStrings) {
var fragmentItem = fragmentItemStrings[i].split('=');
if (fragmentItem.length !== 2) {
continue;
}
fragment[fragmentItem[0]] = fragmentItem[1];
}
var accessToken = fragment["access_token"];
Token Info Endpoint
This endpoint is used for validating implicit grant based access_tokens. Tokens received on the fragment must be validated via this endpoint or they risk being susceptible to the confused deputy problem
URL
https://secure.join.me/api/public/v1/auth/tokenInfo?access_token={access_token}
Method
GETQuery Parameters
Parameter | Value | Description |
---|---|---|
access_token | The access_token that was received in the OAuth flow | Required. |
Response
Parameter | Description |
---|---|
client_id | Client identifier that access_token was granted for |
scope | The space-delimited set of scopes that the user consented to |
expires_at | A timestamp representing when the access_token will expire |